Example of how to implement a search function in ASP.NET with TextBox, Button, and GridView
The following example shows how to create a simple search engine to search for data in a database and display it in a GridView.
Code:
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="search.aspx.cs" Inherits="search" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>Search panel in C# - MdmSoft</title>
<style type="text/css">
.text-search {
height: 24px;
font-family: "Helvetica Neue",helvetica,sans-serif;
font-weight: normal;
color: rgb(0, 143, 213);
font-size: 16px;
padding: 0px 10px;
border-radius: 4px;
background-color: rgb(255, 255, 255);
box-shadow: 0px 1px 2px rgb(81, 184, 228) inset;
border: 1px solid rgb(22, 71, 88);
}
.button-search {
cursor: pointer;
height: 24px;
font-family: "Helvetica Neue",helvetica,sans-serif;
font-weight: bold;
color: white;
font-size: 16px;
padding: 0px 24px;
border-radius: 4px;
background-color: rgb(0, 143, 213);
box-shadow: 0px 1px 2px rgb(81, 184, 228) inset;
text-shadow: 0px 1px 1px rgb(1, 72, 117);
border: 1px solid rgb(22, 71, 88);
}
.button-search:hover {
color: yellow;
}
</style>
<script type="text/javascript">
</script>
</head>
<body>
<form id="formMain" runat="server">
<asp:Panel ID="PanelSearch" runat="server" DefaultButton="ButtonSearch">
<asp:TextBox ID="TextBoxSearch" runat="server"
CssClass="text-search"
Text=""
Width="370" />
<asp:Button ID="ButtonSearch" runat="server"
CssClass="button-search"
Text="Search"
OnClick="ButtonSearch_Click" />
</asp:Panel>
<br />
<div>
<asp:GridView ID="GridViewEmployees" runat="server"
BorderColor="Gray"
BorderStyle="Solid"
BorderWidth="1"
CellPadding="4"
ForeColor="#333333"
GridLines="None"
Width="500">
<AlternatingRowStyle BackColor="White" ForeColor="#284775" />
<EditRowStyle HorizontalAlign="Left" />
<HeaderStyle BackColor="#008ed5" Font-Bold="True" ForeColor="White" />
<RowStyle BackColor="#F7F6F3" ForeColor="#333333" />
</asp:GridView>
<asp:AccessDataSource ID="AccessDataSourceEmployees" runat="server"
DataFile="~/App_Data/Northwind.mdb"></asp:AccessDataSource>
</div>
</form>
</body>
</html>
Code:
using System;
using System.Globalization;
using System.Threading;
using System.Web.UI;
using System.Web.UI.HtmlControls;
public partial class search : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
TextBoxSearch.Focus();
string selectCommand = "SELECT Employees.TitleOfCourtesy AS Title, Employees.LastName AS Surname, Employees.FirstName AS Name, Employees.City " +
"FROM Employees " +
"ORDER BY Employees.LastName";
AccessDataSourceEmployees.SelectCommand = selectCommand;
GridViewEmployees.DataSource = AccessDataSourceEmployees;
GridViewEmployees.DataBind();
}
/// <summary>
/// Search the text in the TextBoxSearch into the database fields and update the GridView contents
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
protected void ButtonSearch_Click(object sender, EventArgs e)
{
if (TextBoxSearch.Text != "")
{
string search = TextBoxSearch.Text;
string selectCommand = "SELECT Employees.TitleOfCourtesy AS Title, Employees.LastName AS Surname, Employees.FirstName AS Name, Employees.City " +
"FROM Employees " +
"WHERE Employees.LastName LIKE'%" + search + "%' OR "+
"Employees.FirstName LIKE'%" + search + "%' OR " +
"Employees.City LIKE'%" + search + "%' " +
"ORDER BY Employees.LastName";
AccessDataSourceEmployees.SelectCommand = selectCommand;
GridViewEmployees.DataSource = AccessDataSourceEmployees;
GridViewEmployees.DataBind();
TextBoxSearch.Focus();
}
}
}
The initial page load the GridView displays all data:
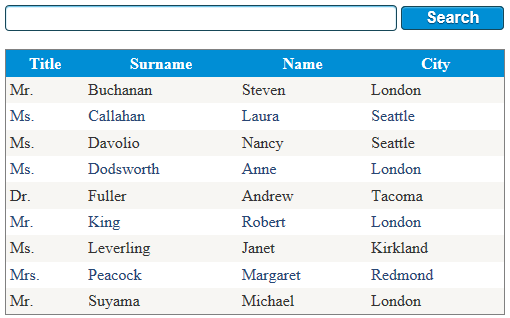
When you type a term in the TextBoxSearch and press enter or click ButtonSearch; the GridView control displays only the records that match the search criteria.
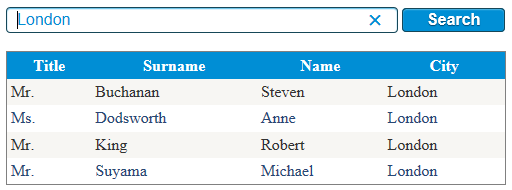
Note: this example has been simplified and should be used only for educational purposes. It does not run any control over the contents of the TextBox. It would be necessary to filter the search string for removing invalid characters, and use the parameters in the SELECT statement.