Use XSLT to transform XML documents into other formats, such as XHTML
Suppose you have an XML document like the one shown below. This document may be the result of exporting a query to a database, or the output of a webservice or other such things.
Code:
<?xml version="1.0" ?>
<books>
<book>
<title>Programming in XML</title>
<author>Marc Vince</author>
<pages>348</pages>
<category>Programming</category>
</book>
<book>
<title>Programming in C#</title>
<author>Loren Gallipyos</author>
<pages>234</pages>
<category>Programming</category>
</book>
<book>
<title>Android</title>
<author>Norman Bert</author>
<pages>419</pages>
<category>Operating Systems</category>
</book>
<book>
<title>Linux</title>
<author>Hurth Francy</author>
<pages>294</pages>
<category>Operating Systems</category>
</book>
<book>
<title>WordPress</title>
<author>Milena Zarch</author>
<pages>364</pages>
<category>CMS</category>
</book>
<book>
<title>Joomla!</title>
<author>Denise Patrics</author>
<pages>163</pages>
<category>CMS</category>
</book>
<book>
<title>jQuery</title>
<author>Teresa Curtis</author>
<pages>349</pages>
<category>Programming</category>
</book>
</books>
If we try to display the file in the browser, we get this result:
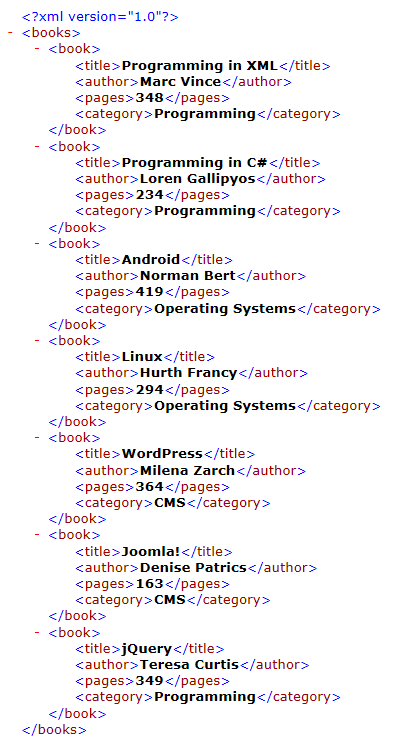
Sometimes it is useful to view the data in a more readable and visually more presentable. To do this we need an XSL file, able to apply a "transformation" to the XML document.
Code:
<?xml version="1.0" encoding="UTF-8"?>
<xsl:stylesheet
version="1.0"
xmlns:xsl="http://www.w3.org/1999/XSL/Transform"
xmlns="http://www.w3.org/1999/xhtml">
<xsl:output method="xml" indent="yes" encoding="UTF-8"/>
<xsl:template match="/books">
<html>
<head> <title>List of books available</title> </head>
<body>
<h1 style="color: #880000;">Books</h1>
<div>
<xsl:apply-templates select="book">
<xsl:sort
data-type="text"
order="ascending"
select="title"
/>
</xsl:apply-templates>
</div>
</body>
</html>
</xsl:template>
<xsl:template match="book">
<div>
<span style="color: #880000;font-size:22px;"><xsl:value-of select="title"/></span>
<br />
Author:<xsl:value-of select="author"/>
<br />
Pages:<xsl:value-of select="pages"/>
<br />
Category: <xsl:value-of select="category"/>
</div>
<br />
</xsl:template>
</xsl:stylesheet>
To apply the transformation of the XML document, you must enter this line of code in the XML file:
Code:
<?xml-stylesheet type="text/xsl" href="books.xsl" ?>
The document will be displayed in the following mode:
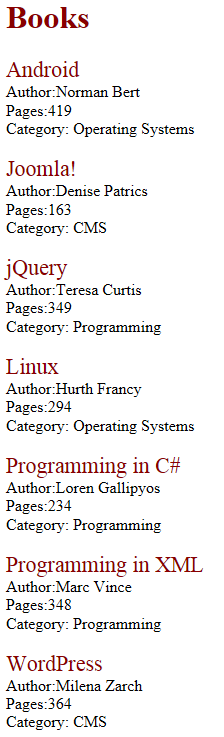