Making a simple form for sending a message with PHP
This example written in PHP allows you to create a simple form to send a message.
Code:
<html>
<style type="text/css">
div.request-information-panel {
background-color:#ccc;
border:1px solid #777;
color:#1B45BA;
font-family:Arial;
font-size:14px;
padding:10px;
width:320px;
}
div.request-information-panel-button {
text-align:right;
}
div.request-information-panel-message {
background-color:#eee;
border:1px solid #777;
color:#333;
font-family:Arial;
font-size:14px;
padding:10px;
width:320px;
}
div.request-information-message {
background-color:lightyellow;
border:1px dotted #777;
color:#333;
font-family:Arial;
font-size:14px;
font-style:italic;
margin-top:10px;
padding:5px;
}
</style>
<body>
<?php
// Checking if all fields are valid
if( isset($_POST["submit"]) &&
isset($_POST["name"]) && $_POST["name"]!="" &&
isset($_POST["company"]) && $_POST["company"]!="" &&
isset($_POST["email"]) && $_POST["email"]!="" &&
isset($_POST["message"]) && $_POST["email"]!="" ) {
// I get field values from $ _POST []
$name = $_POST["name"];
$company = $_POST["company"];
$email = $_POST["email"];
$message = $_POST["message"];
// Print the message of confirm
echo ( '<div class="request-information-panel-message">');
echo ( "Hello $name,<br />");
echo ( "Noi abbiamo ricevuto il tuo messaggio:<br />");
echo ( '<div class="request-information-message">' );
echo ( "$message");
echo ( '</div>');
echo ( '</div>');
// I call the methods (instructions) that I need, for example:
// SendMail( name,company, email, message );
// SaveMessageInDatabase( name,company, email, message );
}
else {
?>
<div class="request-information-panel">
<form action='<?php echo $_SERVER["PHP_SELF"]; ?>' method='post'>
Name:
<br />
<input type='text' name='name' size='50'>
<br /><br>
Company:
<br />
<input type='text' name='company' size='50'>
<br /><br>
Email:
<br />
<input type='text' name='email' size='50'>
<br /><br>
Message:
<br />
<textarea name='message' cols='37' rows='7'></textarea>
<br /><br />
<div class="request-information-panel-button">
<input type='submit' name='submit' value='Send'>
</div>
</form>
</div>
<?php
}
?>
</body>
</html>
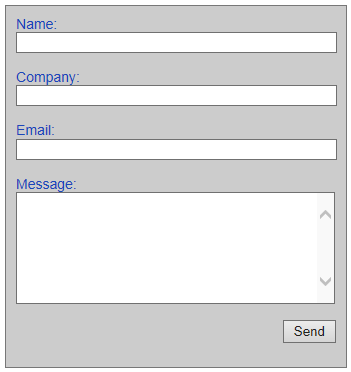
Note: The code is very basic and does not perform any checks on the accuracy/validity of the fields! For optimal use should be added to other control statements.