How to use multidimensional arrays and how to sort a multidimensional array with PHP
The following example shows how to use two-dimensional arrays and how to order the elements.
<style type="text/css">
div.table {
float:left;
font-family: Verdana;
font-size:12px;
margin:15px;
}
table {
border:1px solid #aaa;
border-collapse:collapse;
width:160px;
}
th {
background-color:#777;
color:#fff;
font-size:12px;
text-align:left;
}
td {
background-color:#fff;
font-size:12px;
}
td.ordered {
background-color:#ffffe0;
font-size:12px;
}
</style>
<?php
// Create a multidimensional array
$people = array(
array( "Maria",
"Valery",
"Roxsana",
"Lisa",
"Deborah",
"Barbara",
"Carla",
"Sophie",
"Nadya",
"Enya"
),
array(32,28,35,33,37,35,25,35,28,33)
);
// Print unordered list
echo("<div class='table'>");
echo("Unordered list");
echo("<table border='1' >");
echo("<tr>");
echo("<tr><th>Name</th><th>Age</th></tr>");
for($n=0; $n < count($people[0]); $n++)
{
echo("<tr>");
echo("<td>" . $people[0][$n] . "</td>");
echo("<td>" . $people[1][$n] . "</td>");
echo("</tr>");
}
echo("</table>");
echo("</div>");
// Sort the array by name
array_multisort($people[0],SORT_ASC, SORT_STRING,
$people[1],SORT_NUMERIC);
// Print list sorted by name
echo("<div class='table'>");
echo("List sorted by name");
echo("<table border='1' width='140px'>");
echo("<tr>");
echo("<tr><th>Name</th><th>Age</th></tr>");
for($n=0; $n < count($people[0]); $n++)// as $peopleName)
{
echo("<tr>");
echo("<td class='ordered'>" . $people[0][$n] . "</td>");
echo("<td>" . $people[1][$n] . "</td>");
echo("</tr>");
}
echo("</table>");
echo("</div>");
// Sort the array by age
array_multisort($people[1],SORT_ASC, SORT_NUMERIC,
$people[0],SORT_STRING);
// Print list sorted by age
echo("<div class='table'>");
echo("List sorted by age");
echo("<table border='1' width='140px'>");
echo("<tr>");
echo("<tr><th>Name</th><th>Age</th></th>");
for($n=0; $n < count($people[0]); $n++)// as $peopleName)
{
echo("<tr>");
echo("<td>" . $people[0][$n] . "</td>");
echo("<td class='ordered'>" . $people[1][$n] . "</td>");
echo("</tr>");
}
echo("</table>");
echo("</div>");
?>
Here is the result for the sample:
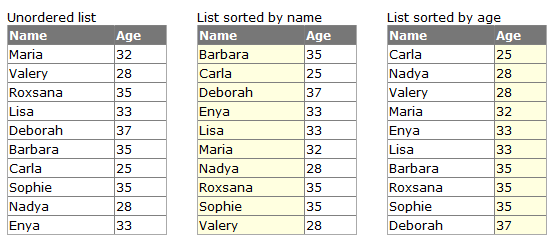