How to generate the six winning numbers to win the Lottery with PHP
The following example shows how to create six random numbers using PHP.
Code:
<style type="text/css">
div.lottery-panel {
background-color:#eee;
border:1px solid #789;
font-family:Verdana;
text-align:center;
width:200px;
}
span.lottery-panel-title {
border-bottom:1px solid green;
color:green;
font-family:Verdana;
font-size:14px;
}
span.lottery-panel-footer {
color:gray;
font-size:12px;
}
table {
margin:10px auto;
width:180px;
}
td {
background-color: orange;
border:1px solid #aaa;
border-radius:14px;
color:black;
font-size:16px;
height:28px;
text-align:center;
width:24px;
}
</style>
<div class="lottery-panel">
<span class="lottery-panel-title">Here's your lucky numbers!</span>
<table border='0'>
<tr>
<?php
// Declare an array of numbers
$numbers = array();
for($n=0;$n<6;) {
// Generate a random number
$r = rand (1, 90);
// Checking if the number is not in the array
if(!in_array( $r, $numbers) ) :
$numbers[$n] = $r;
$n++;
endif;
}
// Order the array in ascending order
sort($numbers);
// Print the numbers drawn
for($n=0;$n<6;$n++) {
if($numbers[$n] == 0)
echo("<td>?</td>");
else
echo("<td>" . $numbers[$n] . "</td>");
}
?>
</tr>
</table>
<span class="lottery-panel-footer">Good luck ;-)</span>
</div>
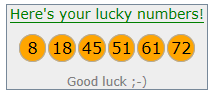
This example is useful to understand some PHP functions:
- - Generate random numbers within a range
- - Check if a number is present in the array
We do not guarantee that you will win the Lottery with this example, but at least you learned something about PHP.