Fill an ASP.NET GridView using data stored in an xml file
XML is a data format very flexible and widely used. With ASP.NET is very simple to insert the data in an xml source into a GridView.
Suppose you have a file like this:
Code:
<?xml version="1.0" ?>
<books>
<book>
<title>Programming in XML</title>
<author>Marc Vince</author>
<pages>348</pages>
<category>Programming</category>
</book>
<book>
<title>Programming in C#</title>
<author>Loren Gallipyos</author>
<pages>234</pages>
<category>Programming</category>
</book>
<book>
<title>Android</title>
<author>Norman Bert</author>
<pages>419</pages>
<category>Operating Systems</category>
</book>
<book>
<title>Linux</title>
<author>Hurth Francy</author>
<pages>294</pages>
<category>Operating Systems</category>
</book>
<book>
<title>WordPress</title>
<author>Milena Zarch</author>
<pages>364</pages>
<category>CMS</category>
</book>
<book>
<title>Joomla!</title>
<author>Denise Patrics</author>
<pages>163</pages>
<category>CMS</category>
</book>
</books>
An ASP.NET page with a GridView:
Code:
<div>
<asp:GridView ID="GridViewBooks" runat="server"
CellPadding="4"
ForeColor="#333333"
GridLines="None"
Width="500">
<AlternatingRowStyle BackColor="White" ForeColor="#284775" />
<EditRowStyle HorizontalAlign="Left" />
<HeaderStyle BackColor="#5D7B9D" Font-Bold="True" ForeColor="White" />
<RowStyle BackColor="#F7F6F3" ForeColor="#333333"/>
</asp:GridView>
</div>
In the code-behind file that you can write code like this:
Code:
try
{
XmlTextReader xmlTextReader = new XmlTextReader(Server.MapPath("~/public/books.xml"));
DataSet dataSetBooks = new DataSet();
dataSetBooks.ReadXml(xmlTextReader);
GridViewBooks.DataSource = dataSetBooks;
GridViewBooks.DataBind();
}
catch (Exception excp)
{
string error = excp.ToString();
// Report error message
}
Here is the result for the sample:
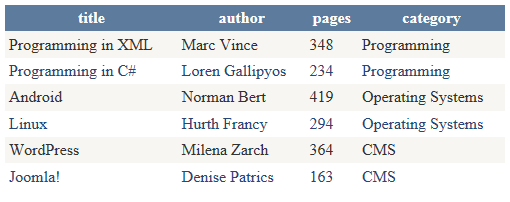