Extremely simple HTML color generator developed with HTML and Javascript
This example allows us to realize in a very simple way a html code generator.
Code:
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>Extremely simple HTML color generator developed with HTML and Javascript - MdmSoft</title>
<style type="text/css">
h1.title {
color: rgb(1, 108, 180);
font-size: 23px;
margin: 0px;
padding: 0px;
}
label {
color: #888;
font-family: Verdana;
font-size: 18px;
letter-spacing: 3px;
padding-right: 30px;
}
table {
background-color: #fff;
border: 1px solid #333;
border-radius: 5px;
padding: 3px;
width: 240px;
}
#red {
color: rgb(255, 0, 0);
}
#green {
color: rgb(0, 255, 0);
}
#blue {
color: rgb(0, 0, 255);
}
#color {
border: 1px solid #333;
background-color: rgb(125, 11, 89);
height: 54px;
width: 70px;
text-align: center;
}
</style>
<script type="text/javascript">
function SetColor() {
var redValue = parseInt(document.getElementById("text-red").value);
var greenValue = parseInt(document.getElementById("text-green").value);
var blueValue = parseInt(document.getElementById("text-blue").value);
var tb = document.getElementById("color");
if (redValue >= 0 && redValue <= 255 &&
greenValue >= 0 && greenValue <= 255 &&
blueValue >= 0 && blueValue <= 255) {
tb.style.backgroundColor = "rgb(" + redValue + ", " + greenValue + ", " + blueValue + ")";
var hexColor = "#" +
decimalToHex(redValue, 2) +
decimalToHex(greenValue, 2) +
decimalToHex(blueValue, 2);
document.getElementById("text-color").value = hexColor.toUpperCase();
}
}
function decimalToHex(d, padding) {
var hex = Number(d).toString(16);
padding = typeof (padding) === "undefined" || padding === null ? padding = 2 : padding;
while (hex.length < padding) {
hex = "0" + hex;
}
return hex;
}
</script>
</head>
<body onload="javascript:SetColor();">
<h1 class="title">HTML Color generator</h1>
<table border="0">
<tr>
<td>
<label id="red" for="Red">Red:</label></td>
<td>
<input id="text-red" type="text" size="1" value="255" onchange="javascript: SetColor();" /></td>
<td id="color" rowspan="3"></td>
</tr>
<tr>
<td>
<label id="green" for="Green">Green:</label></td>
<td>
<input id="text-green" type="text" size="1" value="255" onchange="javascript: SetColor();" /></td>
</tr>
<tr>
<td>
<label id="blue" for="Blue">Blue:</label></td>
<td>
<input id="text-blue" type="text" size="1" value="255" onchange="javascript: SetColor();" />
</td>
</tr>
<tr>
<td>
<label for="Color">HTML</label></td>
<td colspan="2">
<input id="text-color" type="text" size="13" value="" readonly="true" />
</td>
</tr>
</table>
</body>
</html>
Result:
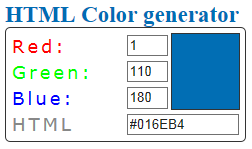