Example of how to use Ajax control Accordation associated with an Access database
This complete sample, shows how to use the Ajax control Accordation associated with a database Access to view invoice information.
Code:
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="AccordationDemo.aspx.cs" Inherits="AccordationDemo" %>
<%@ Register Assembly="AjaxControlToolkit" Namespace="AjaxControlToolkit" TagPrefix="asp" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>Example of how to use Ajax control Accordation associated with an Access database - MdmSoft</title>
<style type="text/css">
body {
font-family: Verdana;
font-size: 12px;
}
.header {
border: 1px solid #777;
color: #333;
margin: 4px;
padding: 2px;
}
.header :hover {
color: #0094ff;
}
.content {
background-color: lightyellow;
border: 1px solid #777;
border-radius: 4px;
margin-left: 20px;
padding: 5px;
}
.content h2.title {
border-bottom: 1px solid #777;
color: #333;
font-size: 12px;
margin: 0;
padding: 0;
}
</style>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:ToolkitScriptManager ID="tsmInvoices" runat="server"></asp:ToolkitScriptManager>
<asp:Accordion ID="AccordionInvoices" runat="server"
ContentCssClass="content"
DataSourceID="AccessDataSourceInvoices"
FadeTransitions="true"
HeaderCssClass="header"
Width="470px">
<HeaderTemplate>
<b>Invoice N°:
<%#DataBinder.Eval(Container.DataItem, "OrderID")%>
of
<%# String.Format("{0:D}",Eval("OrderDate")) %>
</b>
</HeaderTemplate>
<ContentTemplate>
<h2 class="title">Invoice detail: N° <%#DataBinder.Eval(Container.DataItem, "OrderID")%></h2>
<div class="">
<%#DataBinder.Eval(Container.DataItem, "CompanyName")%>
<br />
<%#DataBinder.Eval(Container.DataItem, "Address")%>
<br />
<%#DataBinder.Eval(Container.DataItem, "City")%>
<br />
<%#DataBinder.Eval(Container.DataItem, "PostalCode")%> -
<%#DataBinder.Eval(Container.DataItem, "Country")%>
<br />
Total: <%# String.Format("{0:C}",Eval("ExtendedPrice")) %>
</div>
</ContentTemplate>
</asp:Accordion>
<asp:AccessDataSource ID="AccessDataSourceInvoices" runat="server"></asp:AccessDataSource>
</div>
</form>
</body>
</html>
Code:
using System;
public partial class AccordationDemo : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
AccessDataSourceInvoices.DataFile = Server.MapPath("~/App_Data/Northwind.mdb");
AccessDataSourceInvoices.SelectCommand = @"SELECT TOP 10
OrderID,
OrderDate,
Customers.CompanyName,
Address,
City,
PostalCode,
Country,
ExtendedPrice
FROM Invoices";
AccordionInvoices.DataBind();
}
}
Result:
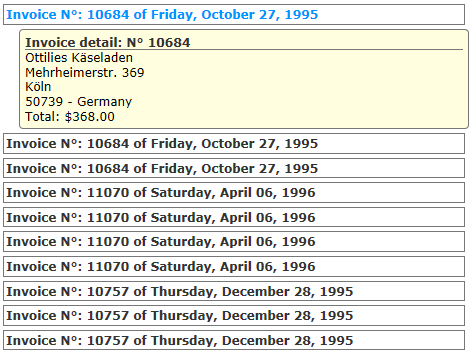
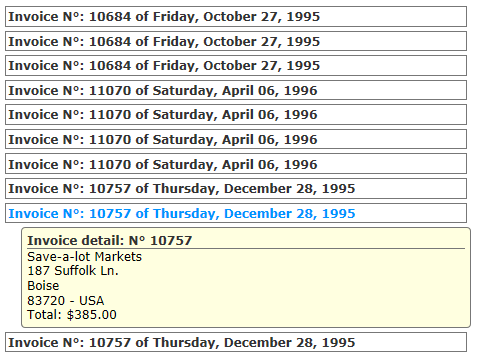