Count the number of occurrences of the characters in a text, with ASP.NET C #
The example allows you to count the number of occurrences of the characters in a text.
Code:
/// <summary>
/// Count the number of occurrences of the characters in a text.
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
protected void ButtonCount_Click(object sender, EventArgs e)
{
if (TextBoxSearch.Text != "")
{
string result = String.Empty;
string phrase = TextBoxSearch.Text;
phrase = phrase.Replace(" ","");
phrase = phrase.ToUpper();
char[] c = phrase.ToCharArray();
Array.Sort(c);
int index = 0;
char[] co = new char[c.Length];
int[] oc = new int[c.Length];
for (int n = 0; n <c.Length; n++)
{
char currentChar = c[n];
if ( IsInArray(co, currentChar) )
{
// Update value occurrence of character
UpdateOccurrence(co, oc, currentChar);
}
else
{
// Add a new value in the array
co[index] = currentChar;
oc[index] = 1;
index++;
}
}
for (int n = 0; n < co.Length; n++)
{
if (oc[n]!=0)
result += co[n] + " = "+oc[n] + "<br />";
}
LabelMessage.Text = result;
TextBoxSearch.Focus();
}
}
/// <summary>
/// Update value occurrence of character
/// </summary>
/// <param name="co"></param>
/// <param name="oc"></param>
/// <param name="currentChar"></param>
private void UpdateOccurrence(char[] co, int[] oc, char currentChar)
{
for (int n = 0; n < co.Length; n++)
{
if (currentChar == co[n])
{
oc[n] = oc[n] + 1;
}
}
}
/// <summary>
/// Check whether the character is in the matrix
/// </summary>
/// <param name="co"></param>
/// <param name="currentChar"></param>
/// <returns></returns>
private bool IsInArray(char[] co, char currentChar)
{
bool isInArray = false;
for (int n = 0; n < co.Length; n++)
{
if (currentChar == co[n])
isInArray = true;
}
return isInArray;
}
Result of this example:
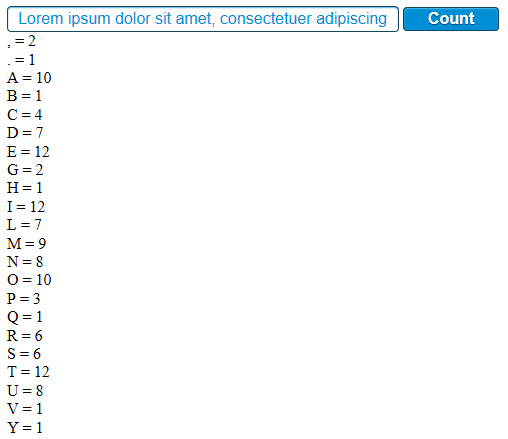