An example of using ASP.NET Calendar control inside an UpdatePanel
The following example shows how to use the control ASP.NET Calendar control to select a date. The Calendar was inserted inside an UpdatePanel in order to make updates without having to reload the entire page.
Code:
<asp:ScriptManager ID="ScriptManagerCalendar" runat="server">
</asp:ScriptManager>
<asp:UpdatePanel ID="UpdatePanelCalendar" runat="server">
<ContentTemplate>
<asp:Label ID="LabelDate" runat="server" Text="Date:"></asp:Label>
<asp:TextBox ID="TextBoxDate" runat="server" Font-Size="Small"
Height="10"
ReadOnly="true"
Width="80px" ></asp:TextBox>
<asp:ImageButton ID="ImageButtonCalendar" runat="server"
ImageUrl="~/images/calendar.gif"
OnClick="ImageButtonCalendar_Click" />
<asp:Calendar ID="Calendar" runat="server"
BackColor="#FFFFCC"
BorderColor="#FFCC66"
BorderWidth="1px"
DayNameFormat="Shortest"
Font-Names="Verdana"
Font-Size="8pt"
ForeColor="#663399"
Height="200px"
ShowGridLines="True"
Visible="false"
Width="220px"
OnSelectionChanged="Calendar_SelectionChanged">
<DayHeaderStyle BackColor="#FFCC66" Font-Bold="True" Height="1px" />
<NextPrevStyle Font-Size="9pt" ForeColor="#FFFFCC" />
<OtherMonthDayStyle ForeColor="#CC9966" />
<SelectedDayStyle BackColor="#CCCCFF" Font-Bold="True" />
<SelectorStyle BackColor="#FFCC66" />
<TitleStyle BackColor="#990000" Font-Bold="True" Font-Size="9pt" ForeColor="#FFFFCC" />
<TodayDayStyle BackColor="#FFCC66" ForeColor="White" />
</asp:Calendar>
</ContentTemplate>
</asp:UpdatePanel>
In the code-behind
Code:
/// <summary>
/// View the Calendar control
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
protected void ImageButtonCalendar_Click(object sender, ImageClickEventArgs e)
{
Calendar.Visible = true;
}
/// <summary>
/// Gets the selected date on the Calendar control and writes in TextBox
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
protected void Calendar_SelectionChanged(object sender, EventArgs e)
{
DateTime dt = Calendar.SelectedDate;
TextBoxDate.Text = dt.ToString("dd/MM/yyyy");
Calendar.Visible = false;
}
Here is the result of the example:
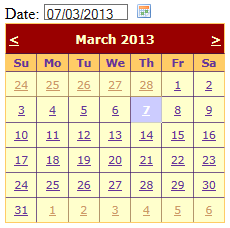